Quickstart
Unreal Engine provides great multiplayer capabilities out of the box. We'll use Unreal's built-in networking to build and deploy a server-authoritative multiplayer game on top of Rivet.
This guide was built with Unreal v5.2 but should work for all Unreal 5 versions. If you run in to issues, please reach out to us on Discord.
Goals
- Deploy & distribute a multiplayer Unreal Engine dedicated game server to Rivet
- Build a basic menu screen for a multiplayer game
- How to test multiplayer games locally
Prerequisites
- Docker
- Unreal Engine 5 GitHub access
- Unreal Engine 5
- This guide was written using Unreal Engine 5.2.1.
- About 60 GB of free space (for Unreal Engine Linux build)
FAQ
Step 1: Setup Unreal Engine project
We'll use the third-person template from Unreal Engine. All the components come with networking out of the box. These steps also apply to any other Unreal Engine games.
Heads up!
If you're following this guide on an existing project, ensure that your project is version controlled before making any changes.
Authenticate with GitHub Container Registry
We need to authenticate with the GitHub Container Registry (GHCR) to be able to pull the Unreal Engine Docker image.
- Make sure you already have Unreal Engine GitHub Access here.
- Authenticate with these instructions.
Create project from template (or bring your own project)
- Create a third-person template with the C++ version. We'll create a guide for using Blueprints instead of C++ soon.
- Set Quality Preset, Starter Content, and Raytracing to anything.
- Click Create.
- Open the Visual Studio project by clicking Tools > Open Visual Studio.
Add server target
We need to add a target so you can compile your game as a dedicated server to run on Rivet.
Create a file at Source/MyProjectServer.Target.cs
with the following. Replace MyProject
with your project's name.
Step 2: Integrate Rivet
Install Rivet CLI
Heads up!
Validate that the version of your CLI is 1.3.4
by running rivet --version
.
Initiate project
Run the following in your project's directory and follow the steps.
Add dependency
Add Rivet to PrivateDependencyModuleNames
in your project Source/MyProject/MyProject.Build.cs
. For example:
Update AMyProjectGameMode
class
We will update the game mode code to connect player connect & disconnect events to Rivet.
Update the the game MyProjectGameMdoe
header & source files to look like the following. Replace MyProject
with your project name.
Restart Unreal Engine for the new C++ header to take effect.
Step 3: Build game menu UI
We will build a simple menu UI that will allow players to connect to a server. This will be the first thing players see when they start the game. We will also update the default map to be the menu UI instead of the game world.
Create the menu UI world
- Create a new level in File -> New Level...
- Select Empty Level
- Click File -> Save Current Level or press Ctrl + S. Save in All/Content/Third Person/Maps and name it Entry.
- Change the game mode by clicking the Blueprint dropdown and then clicking World Override -> Game Mode: Not overridden! -> Select GameModeBase Class -> GameModeBase - This will remove the game mode logic to create a pure menu screen
Create the menu widget
- Open the Content Drawer
- Open All/Content/ThirdPerson/UI
- You may have to create this folder
- Right-click in the content area and click User Interface -> Widget Blueprint
- Click User Widget
- Rename it to EntryWidget
Design menu widget
- Open the EntryWidget you created
- Construct the following design:
Write widget blueprint to connect to server when button is clicked
- Create a development token by running the following command and copying the token. It should look like
dev_staging.XXXX
. Save this for the next step.Command Line - Open the Blueprint code for the EntryWidget you created by clicking Graph in the top right corner
- Replace
MY_TOKEN
with the token you just created.
- Replace
- Construct the following Blueprint:
- Press Compile
This widget can now be used to connect a player to a server.
Add the menu widget to the entry level
- Open the level Blueprint by clicking the Blueprint dropdown and then clicking Open Level Blueprint
- Construct the following Blueprint:
- Press Compile
Update the default map
Now that we have a new Entry map for the UI, we need to make it the default map.
- Open Edit -> Project Settings...
- Click on Maps & Modes under Projects in the sidebar
- You can also search for "map" in the search bar
- Change Game Default Map to /Game/ThirdPerson/Maps/Entry
- Change Advanced > Server Default Map to /Game/ThirdPerson/Maps/ThirdPersonMap
Set up play configuration for testing multiplayer
We will configure the play settings to test the game with Rivet locally in your editor.
-
Press the three dots next to the play button and click Advanced Settings to open the Play In settings.
-
Scroll down to Multiplayer Options. Update the following settings:
- Launch Separate Server: False
- Play Net Mode: Play Standalone
- Run Under One Process: True
-
Update the number of players to at least 2.
Might be useful!
Learn more about different ways to run your multiplayer game in Unreal Engine here.
Test the game server locally
Run server
This will build & run your game server locally and run on port 7777. You must re-run this command whenever you change your server code.
Run client
- Switch to the main editor
- Validate that the Entry level is open
- Click Play
- Click Connect in each of the game instances created
- You should be able to see each player running around in sync
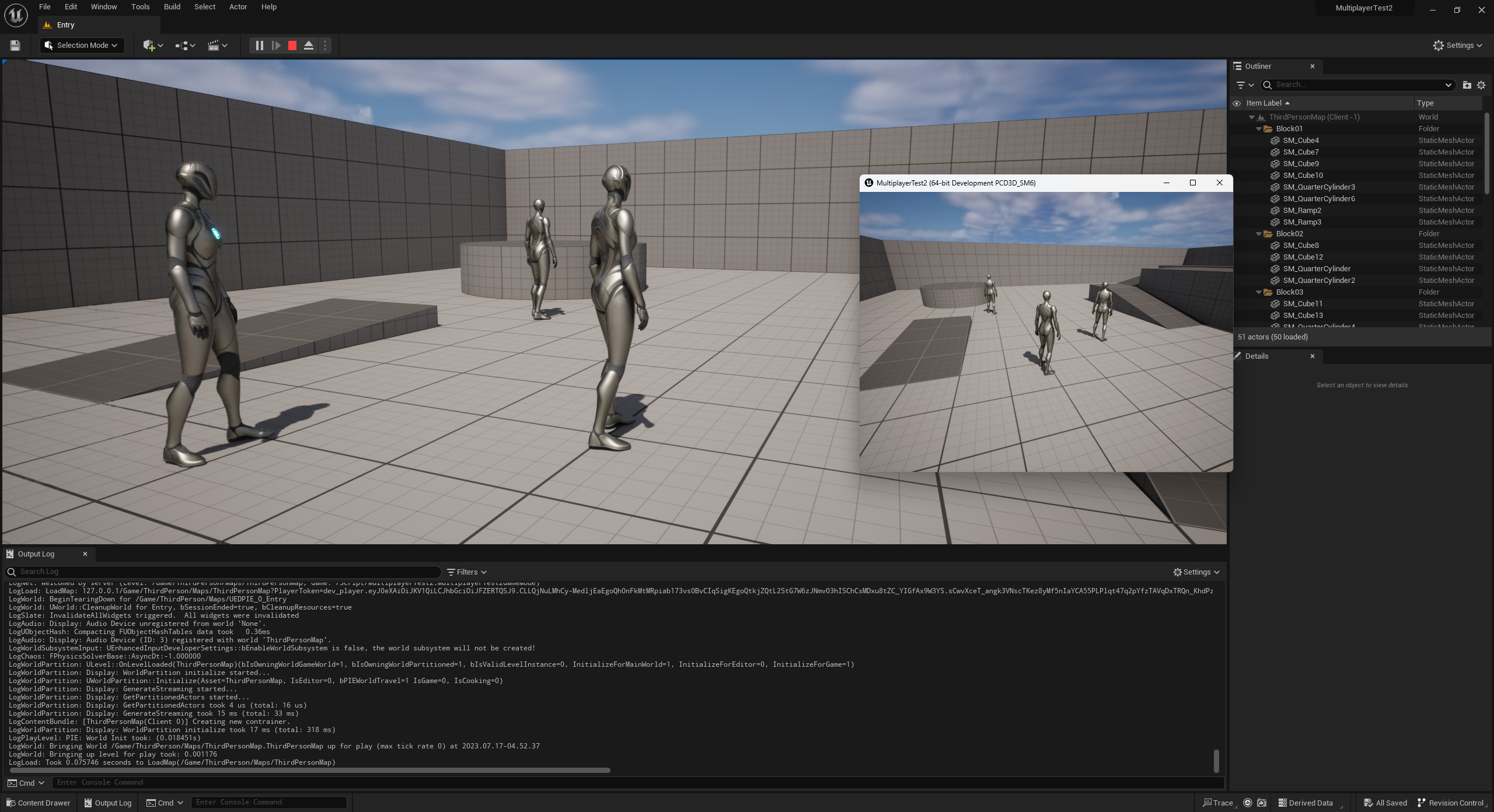
This is using the Rivet development token to mock production APIs. This lets you test with production APIs while still using your local machine.
Step 4: Deploy to Rivet & distribute game
Deploy to Rivet
In the root of the project, run the following command:
This will deploy your project to your game's production environment.
Test with remote game server locally
Now that your game is deployed to Rivet, you can test using a production environment token.
- Create a new Public Token under your environment's Tokens tab
- Update the token in the Entry widget blueprint where you set
MY_TOKEN
in the previous step. - Play the project and click your Connect button. Check that you are able to see the other player run around.
Your game is now up and running on Rivet!
You can see the lobbies in the Rivet dashboard. These will automatically scale up and down as players come and go.
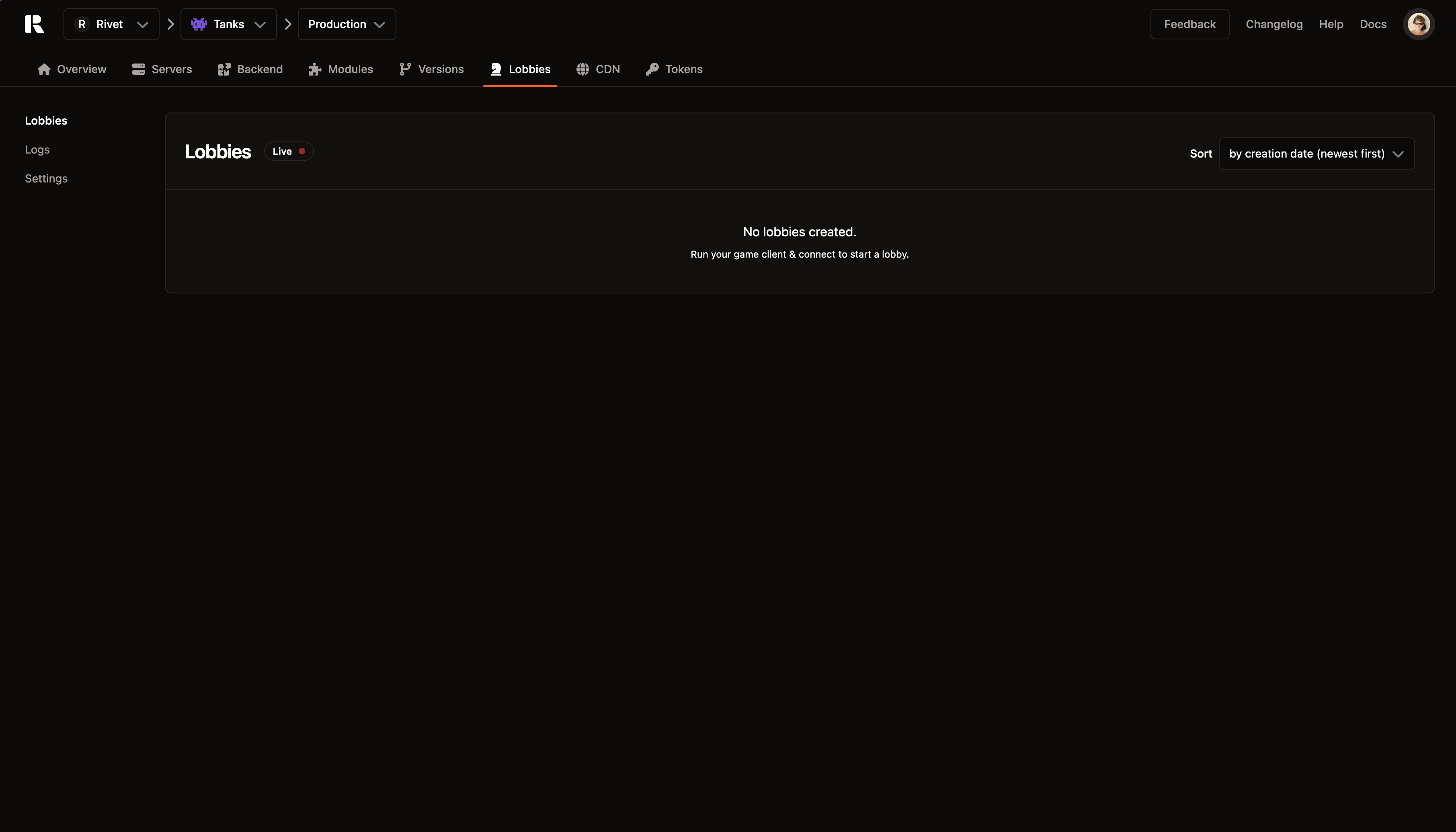
Build & distribute client
You can now build & distribute the game client as you normally would.
Make sure to change Rivet Token to the correct Public Token for your environment when distributing builds, like we did in a previous step.
You can also use Unreal's config files to configure the token.